Conversation Controls
Nuance Messaging SDK comes with few custom Converstation views which you can use in your App layout.
Plain Bubble View
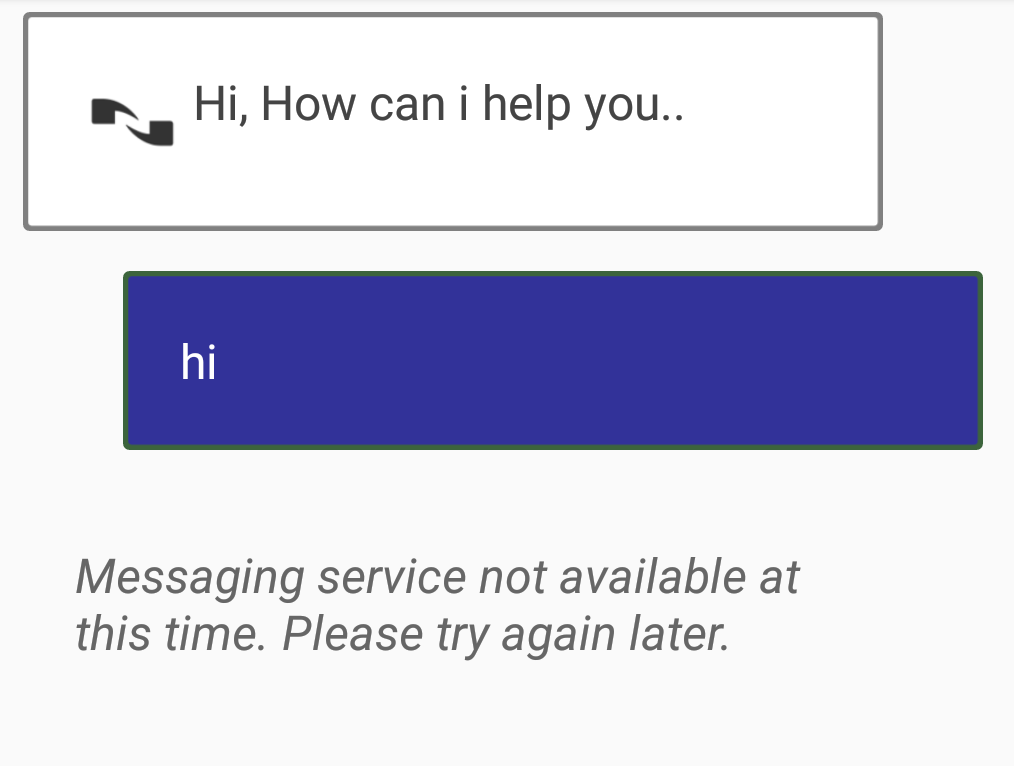
Show/Hide Icon
<bool name="useIconInBubbles">true/false</bool>Style Properties
There are various properties that can be set to look and feel of plain bubble view.
Name | type | description |
---|---|---|
agentIcon | reference | Set the drawable to be displayed in the agent message bubble. |
customerIcon | reference | set the drawable to be displayed in the agent message bubble. |
agentBubbleColor | color | Agent message bubble background color. |
customerBubbleColor | color | Customer message bubble background color. |
systemBubbleColor | color | System message bubble background color. |
typingBubbleColor | color | Typing message bubble background color. |
agentTextColor | color | Agent message text color. |
customerTextColor | color | Customer message text color. |
systemTextColor | color | System message text color. |
typingTextColor | color | Typing message text color. | agentTextStyle | enum|integer | Set the agent message text style. |
customerTextStyle | enum|integer | Set the customer message text style |
systemTextStyle | enum|integer | Set the system message text style |
typingTextStyle | enum|integer | Set the typing message text style |
agentStrokeColor | color | Set the agent message bubble border color. |
customerStrokeColor | color | Set the customer message bubble border color. |
customerStrokeSize | dimention | Set the customer message bubble border width. |
customerCornerRadius | dimention | Set the customer message bubble border radius. |
agentStrokeSize | dimention | Set the agent message bubble border width. |
agentCornerRadius | dimention | Set the agent message bubble border radius. |
typingStrokeSize | dimention | Set the typing message bubble border width. |
typingCornerRadius | dimention | Set the typing message bubble border radius. |
typingStrokeColor | color | Set the typing message bubble border color. |
systemStrokeSize | dimention | Set the system message bubble border width. |
systemCornerRadius | dimention | Set the system message bubble border radius. |
systemStrokeColor | color | Set the system message bubble border color. |
agentMarginLeft | dimention | Set the agent message bubble left margin. |
agentMarginTop | color | Set the agent message bubble top margin. |
agentMarginRight | dimention | Set the agent message bubble right margin. |
agentMarginBottom | dimention | Set the agent message bubble bottom margin. |
customerMarginLeft | dimention | Set the customer message bubble left margin. |
customerMarginTop | color | Set the customer message bubble top margin. |
customerMarginRight | dimention | Set the customer message bubble right margin. |
customerMarginBottom | dimention | Set the customer message bubble bottom margin. |
typingMarginLeft | dimention | Set the typing message bubble left margin. |
typingMarginTop | color | Set the typing message bubble top margin. |
typingMarginRight | dimention | Set the typing message bubble right margin. |
typingMarginBottom | dimention | Set the typing message bubble bottom margin. |
systemMarginLeft | dimention | Set the system message bubble left margin. |
systemMarginTop | color | Set the system message bubble top margin. |
systemMarginRight | dimention | Set the system message bubble right margin. |
systemMarginBottom | dimention | Set the system message bubble bottom margin. |
agentBubblePadding | dimention | Set the agent message bubble padding size. |
customerBubblePadding | color | Set the customer message bubble padding size. |
typingBubblePadding | dimention | Set the typing message bubble padding size. |
systemBubblePadding | dimention | Set the system message bubble padding size. |
agentBubbletextSize | dimention | Set the agent message text size. |
customerBubbletextSize | color | Set the customer message text size. |
typingBubbletextSize | dimention | Set the typing message text size. |
systemBubbletextSize | dimention | Set the system message text size. |
Public Methods
Along with above styling attributes , you must use below public method to actually set the text in the bubble. This is typically done in the RecyclerViewAdapter.onBindViewHolder
-
public void setBubbleTypeTextDirection(BubbleType type, String text, String name, ArrowDirection direction)
BubbleType type: Following are the values for bubble type
BubbleType.AGENT_MESSAGE
BubbleType.CUSTOMER_MESSAGE
BubbleType.TYPING_MESSAGE
BubbleType.SYSTEM_MESSAGE
String text: name to be displayed in the bubble.
String text: text message to be displayed in the bubble.
ArrowDirection direction: For Plain Bubble View ,arrow direction should be set to ArrowDirection.NONE
<android.support.v7.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
app:cardBackgroundColor="@android:color/transparent"
app:cardElevation="0dp"
app:cardPreventCornerOverlap="false"
app:cardMaxElevation="0dp"
>
<com.nuance.chatui.BubbleChatLine
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/message_list_item"
app:agentBubbleColor="#7997a1"
app:agentBubblePadding="20dp"
app:agentStrokeColor="#222b2e"
app:customerBubbleColor="#b5dbe8"
app:customerStrokeColor="#222b2e"
app:customerBubblePadding="20dp">
</com.nuance.chatui.BubbleChatLine>
Arrow Bubble View
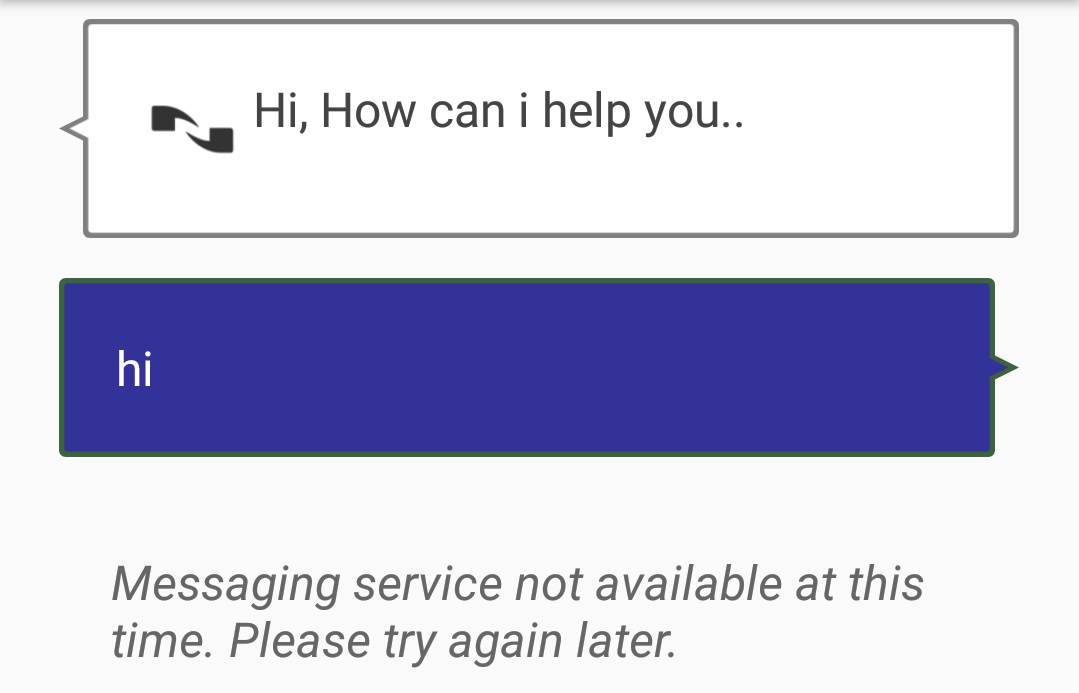
To show arrow in Plain Bubble view. Add the following to the resource file
<bool name="useArrowInBubbles">true</bool>Public Methods
Along with the styling attributes , you must use below public method to actually set the text and arrow direction of the bubble. This is typically done in the RecyclerViewAdapter.onBindViewHolder
-
public void setBubbleTypeTextDirection(BubbleType type, String text, String name, ArrowDirection direction)
BubbleType type: Following are the values for bubble type
BubbleType.AGENT_MESSAGE
BubbleType.CUSTOMER_MESSAGE
BubbleType.TYPING_MESSAGE
BubbleType.SYSTEM_MESSAGE
String name: name to be displayed in the bubble.
String text: text message to be displayed in the bubble.
ArrowDirection direction: Following arrow directions are available to set.
ArrowDirection.LEFT
ArrowDirection.RIGHT
ArrowDirection.TOP
ArrowDirection.BOTTOM
ArrowDirection.LEFT_TOP
ArrowDirection.LEFT_BOTTOM
ArrowDirection.TOP_LEFT
ArrowDirection.TOP_RIGHT
ArrowDirection.BOTTOM_LEFT
ArrowDirection.BOTTOM_RIGHT
ArrowDirection.RIGHT_TOP
ArrowDirection.RIGHT_BOTTOM
ArrowDirection.NONE
@Override
public void onBindViewHolder(final ViewHolder holder, int position) {
Message message = holder.mItem = mValues.get(position);
if(message.getType() == BubbleType.AGENT_MESSAGE){
holder.bubbleChatLine.setBubbleTypeTextDirection(message.getType(),message.getMessageText(), message.getName(), ArrowDirection.TOP_LEFT);
} else if(message.getType() == BubbleType.CUSTOMER_MESSAGE) {
holder.bubbleChatLine.setBubbleTypeTextDirection(message.getType(),message.getMessageText(), message.getName(), ArrowDirection.BOTTOM_RIGHT);
} else if(message.getType() == BubbleType.TYPING_MESSAGE || message.getType() == BubbleType.SYSTEM_MESSAGE) {
holder.bubbleChatLine.setBubbleTypeTextDirection(message.getType(),message.getMessageText(), message.getName(), ArrowDirection.NONE);
}
}
Speech Bubble View

Show/Hide Speech Icon
<bool name="useSpeechChatLine">true/false</bool>Style Properties
There are various properties that can be set to look and feel of Speech bubble view.
Name | type | description |
---|---|---|
agentSpeechIcon | reference | Set the drawable to be displayed in the agent message bubble. |
customerSpeechIcon | reference | set the drawable to be displayed in the agent message bubble. |
agentSpeechColor | color | Agent message bubble background color. |
customerSpeechColor | color | Customer message bubble background color. |
systemSpeechColor | color | System message bubble background color. |
typingSpeechColor | color | Typing message bubble background color. |
agentSpeechTextColor | color | Agent message text color. |
customerSpeechTextColor | color | Customer message text color. |
systemSpeechTextColor | color | System message text color. |
typingSpeechTextColor | color | Typing message text color. | agentSpeechTextStyle | enum|integer | Set the agent message text style. |
customerSpeechTextStyle | enum|integer | Set the customer message text style |
systemSpeechTextStyle | enum|integer | Set the system message text style |
typingSpeechTextStyle | enum|integer | Set the typing message text style |
customerSpeechStrokeColor | color | Set the agent message bubble border color. |
agentSpeechStrokeColor | color | Set the customer message bubble border color. |
customerSpeechStrokeSize | dimention | Set the customer message bubble border width. |
customerSpeechCornerRadius | dimention | Set the customer message bubble border radius. |
agentSpeechStrokeSize | dimention | Set the agent message bubble border width. |
agentSpeechCornerRadius | dimention | Set the agent message bubble border radius. |
typingSpeechStrokeSize | dimention | Set the typing message bubble border width. |
typingSpeechCornerRadius | dimention | Set the typing message bubble border radius. |
typingSpeechStrokeColor | color | Set the typing message bubble border color. |
systemSpeechStrokeSize | dimention | Set the system message bubble border width. |
systemSpeechCornerRadius | dimention | Set the system message bubble border radius. |
systemSpeechStrokeColor | color | Set the system message bubble border color. |
agentSpeechMarginLeft | dimention | Set the agent message bubble left margin. |
agentSpeechMarginTop | color | Set the agent message bubble top margin. |
agentSpeechMarginRight | dimention | Set the agent message bubble right margin. |
agentSpeechMarginBottom | dimention | Set the agent message bubble bottom margin. |
customerSpeechMarginLeft | dimention | Set the customer message bubble left margin. |
customerSpeechMarginTop | color | Set the customer message bubble top margin. |
customerSpeechMarginRight | dimention | Set the customer message bubble right margin. |
customerSpeechMarginBottom | dimention | Set the customer message bubble bottom margin. |
typingSpeechMarginLeft | dimention | Set the typing message bubble left margin. |
typingSpeechMarginTop | color | Set the typing message bubble top margin. |
typingSpeechMarginRight | dimention | Set the typing message bubble right margin. |
typingSpeechMarginBottom | dimention | Set the typing message bubble bottom margin. |
systemSpeechMarginLeft | dimention | Set the system message bubble left margin. |
systemSpeechMarginTop | color | Set the system message bubble top margin. |
systemSpeechMarginRight | dimention | Set the system message bubble right margin. |
systemSpeechMarginBottom | dimention | Set the system message bubble bottom margin. |
agentSpeechPadding | dimention | Set the agent message bubble padding size. |
customerSpeechPadding | color | Set the customer message bubble padding size. |
typingSpeechPadding | dimention | Set the typing message bubble padding size. |
systemSpeechPadding | dimention | Set the system message bubble padding size. |
agentSpeechtextSize | dimention | Set the agent message text size. |
customerSpeechtextSize | color | Set the customer message text size. |
typingSpeechtextSize | dimention | Set the typing message text size. |
systemSpeechtextSize | dimention | Set the system message text size. | agentIconStrokeSize | dimention | Set the size of the border around agent Speech Icon. |
agentIconStrokeColor | color | Set the color of the border around agent Speech Icon |
customerIconStrokeSize | dimention | Set the size of the border around customer Speech Icon |
customerIconStrokeColor | color | Set the color of the border around customer Speech Icon |
Public Methods
Along with above styling attributes , you must use below public method to actually set the text in the bubble. This is typically done in the RecyclerViewAdapter.onBindViewHolder
-
public void setSpeechTypeText(BubbleType type, String text)
BubbleType type: Following are the values for bubble type
BubbleType.AGENT_MESSAGE
BubbleType.CUSTOMER_MESSAGE
BubbleType.TYPING_MESSAGE
BubbleType.SYSTEM_MESSAGE
String text: text message to be displayed in the bubble.
<android.support.v7.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
app:cardBackgroundColor="@android:color/transparent"
app:cardElevation="0dp"
app:cardPreventCornerOverlap="false"
app:cardMaxElevation="0dp"
>
<com.nuance.chatui.SpeechChatLine
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/message_list_item"
app:agentSpeechIcon="@drawable/agentSpeechicon"
app:customerSpeechIcon="@drawable/customerSpeechicon"
app:agentSpeechStrokeColor="#222b2e"
app:customerSpeechColor="#b5dbe8"
app:customerSpeechStrokeColor="#222b2e"
app:customerSpeechPadding="20dp">
</com.nuance.chatui.SpeechChatLine>