Quick Start Guide
Prerequisites
To use the Nuance Messaging SDK, you will need the following.
- Nuance Messaging AAR library for Android
- Gradle Build System
Getting the Nuance Messaging Android SDK from artifactory repository
Configure below files to point to Nuance maven repository.
-
gradle.properties
Feed name for prod is nuance-sdk-android and for beta is nuance-sdk-android-beta.
nuanceArtifactoryFeedName=<FEED_NAME> nuanceArtifactoryOrgUrl=https://pkgs.dev.azure.com/nuance-ent-rd-fe/sdk-android/_packaging nuanceArtifactoryAccessToken=<YOUR_ACCESS_TOKEN>
-
build.gradle-project level
Minimum Gradle version is 6.7.1 and build tools version is 4.2.0
allprojects { repositories { maven { String nuanceArtifactoryUrl = project.properties["nuanceArtifactoryOrgUrl"]+"/"+project.properties["nuanceArtifactoryFeedName"]+"/maven/v1" url nuanceArtifactoryUrl credentials { username project.properties["nuanceArtifactoryFeedName"] password project.properties["nuanceArtifactoryAccessToken"] } } } }
Getting the SDK as an AAR from artifactory repository
We encourage you to use Dependency Manager to manage Nuance Messaging SDK dependency in your project. However, downloading Android artefacts are simple in Azure Devops.
1. Go to Azure Devops Home https://dev.azure.com/nuance-ent-rd-fe/
2. Navigate to Artifacts from the left menu.
3. Select nuance.enterprise.nsdk:nuancemessaging feed.
4. On the next step you will be able to download the aar file from the files section on the bottom.
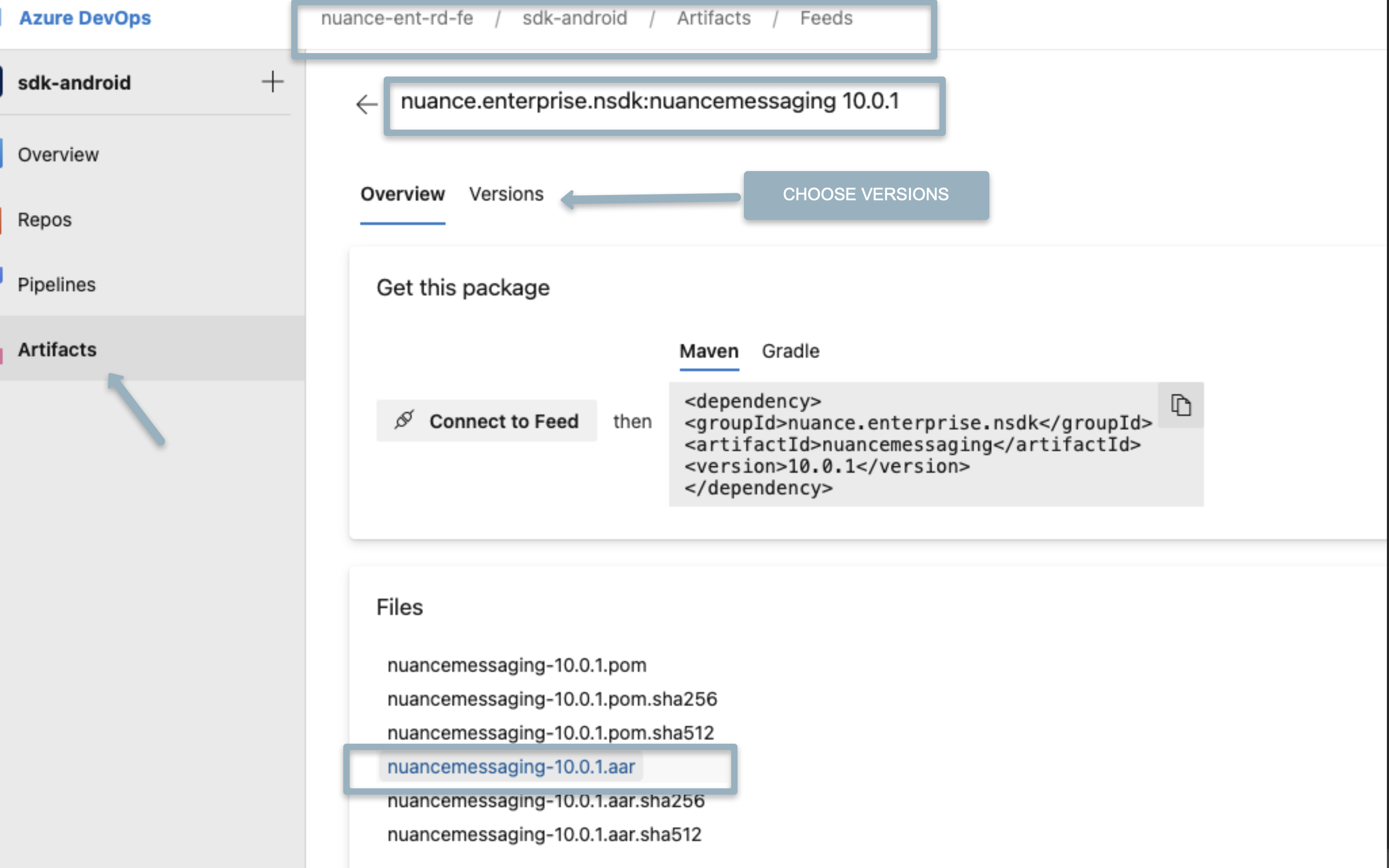
Getting the zipped Nuance Messaging Android SDK
This distribution mechanism is going to be obsolete soon. We recommend to use maven artifactory.
Contact your Nuance Client Services Manager to get the Android SDK package. The package includes the following elements.
-
.aar file
A module that must be added to your project.
-
ExampleApps
Several example apps that demonstrate the features and how to use them.
-
readMe.txt
Contains information about the example app and the features it uses.
Configuring the SDK from artifactory repository
Follow these steps to configure the project settings and connect to the SDK.
-
Add nuance module dependencies to the build.gradle of your app :
ext { nuanceVersion = "10.3.1" } dependencies { implementation "nuance.enterprise.nsdk:nuancemessaging:$nuanceVersion" implementation "nuance.enterprise.nsdk:nuanpreview:$nuanceVersion" implementation "nuance.enterprise.nsdk:translator:$nuanceVersion" implementation "nuance.enterprise.nsdk:translatorpersona:$nuanceVersion" implementation "nuance.enterprise.nsdk:wakeup:$nuanceVersion" }
-
Add nuance module dependencies to the build.gradle of your app :
implementation 'androidx.appcompat:appcompat:1.2.0' implementation 'com.android.volley:volley:1.2.0' implementation 'androidx.recyclerview:recyclerview:1.2.0' implementation 'androidx.legacy:legacy-support-v4:1.0.0' implementation 'com.google.android.material:material:1.3.0' implementation 'org.greenrobot:eventbus:3.1.1' implementation 'com.google.android:flexbox:1.0.0' implementation 'androidx.constraintlayout:constraintlayout:2.0.4' implementation 'androidx.cardview:cardview:1.0.0' implementation 'com.github.bumptech.glide:glide:4.12.0'
Configuring the zipped SDK
This distribution mechanism is going to be obsolete soon. We recommend to use maven artifactory.
Follow these steps to configure the project settings and connect to the SDK.
-
Import the nuancemessaging.aar module into your project:
- Right click on App root folder name Open Module Settings → Add Module.
- Choose Import JAR/.AAR Package from the given options.
- Navigate to the folder where you extracted the SDK Package. Then, navigate to the nuancemessaging.aar module, and click Finish.
-
Add the following lines to the build.gradle of your app :
implementation project(path: ':nuancemessaging')
-
Add nuance module dependencies to the build.gradle of your app :
// Third Party libraries implementation 'com.android.volley:volley:1.2.0' implementation 'org.greenrobot:eventbus:3.1.1' implementation 'com.github.bumptech.glide:glide:4.12.0' // Android libraries implementation 'androidx.appcompat:appcompat:1.2.0' implementation 'androidx.recyclerview:recyclerview:1.2.0' implementation 'androidx.legacy:legacy-support-v4:1.0.0' implementation 'com.google.android.material:material:1.3.0' implementation 'com.google.android:flexbox:1.0.0' implementation 'androidx.constraintlayout:constraintlayout:2.0.4' implementation 'androidx.cardview:cardview:1.0.0'
Third Party Licenses
com.android.volley:volley:1.2.0 | ![]() |
org.greenrobot:eventbus:3.1.1 | ![]() |
com.github.bumptech.glide:glide:4.12.0 | ![]() |
Configuring Translator SDK
Follow these steps to have ASR (Automatic Speech Recongnition) and TTS (Text to speach) functionality in your Application.
-
Import the translator.aar and translatorpersona module into your project:
- In the Android Studio menu bar, select: File → New → Import Module.
- Choose Import JAR/.AAR Package from the given options.
- Navigate to the folder where you extracted the SDK Package. Then, navigate to the nuancemessaging.aar module, and click Finish.
-
Add the following lines to the build.gradle of your app :
implementation("com.squareup.okhttp3:okhttp:4.9.3") api project(':translator') api project(':translatorpersona')
Initialization and Launch
The messaging module must be initialized before any of its components start executing the lifecycle methods. It is recommended that you initialize the SDK once in your application class. The Nuance Messaging module is built by using various Android elements such as Service, Activity and Broadcast Receiver.
To initialize the module, invoke the initialize method in the NuanMessaging class.
NuanMessaging
- initialize(Context context, String dc, String clientID, String clientSecret, String tagServerName)
- initialize(Context context, String dc, String clientID, String clientSecret, String tagServerName, RequestQueue requestQueue)
- initialize(Context context, String dc, String clientID, String clientSecret, String tagServerName, String fcmToken)
- initialize(Context context, String dc, String clientID, String clientSecret, String tagServerName, RequestQueue requestQueue, String fcmToken)
clientID | Pass the Nuance-provided clientID which will be used to identify your messaging instance. |
clientSecret | Pass the Nuance-provided clientSecret code. |
dc | The name of the data center where your messaging session is processed. |
tagServerName | Request for Nuance to provide the name of the tagserver that will handle your messages. |
RequestQueue | Instance of Volley.RequestQueue. If this is not provided, NuanMessaging will create a new volley instance. |
fcmToken | If a device registration token is provided, the SDK can register to receive PUSH messages from the Nuance Messaging System. |
public class Application extends android.app.Application { NuanMessaging nuanMessaging; String clientID; String clientSecret; @Override public void onCreate() { super.onCreate(); nuanMessaging = NuanMessaging.getInstance(); clientID = "Your client Id"; cientSecret = "your client secret" nuanMessaging.initialize(this,"west","ceapiClientId", "ceapiClientSecret","bestbrands"); } }
Messaging Window Launch/Restore Flow diagram
Application needs to follow the below code path when user launches or navigate to different screen within the App.
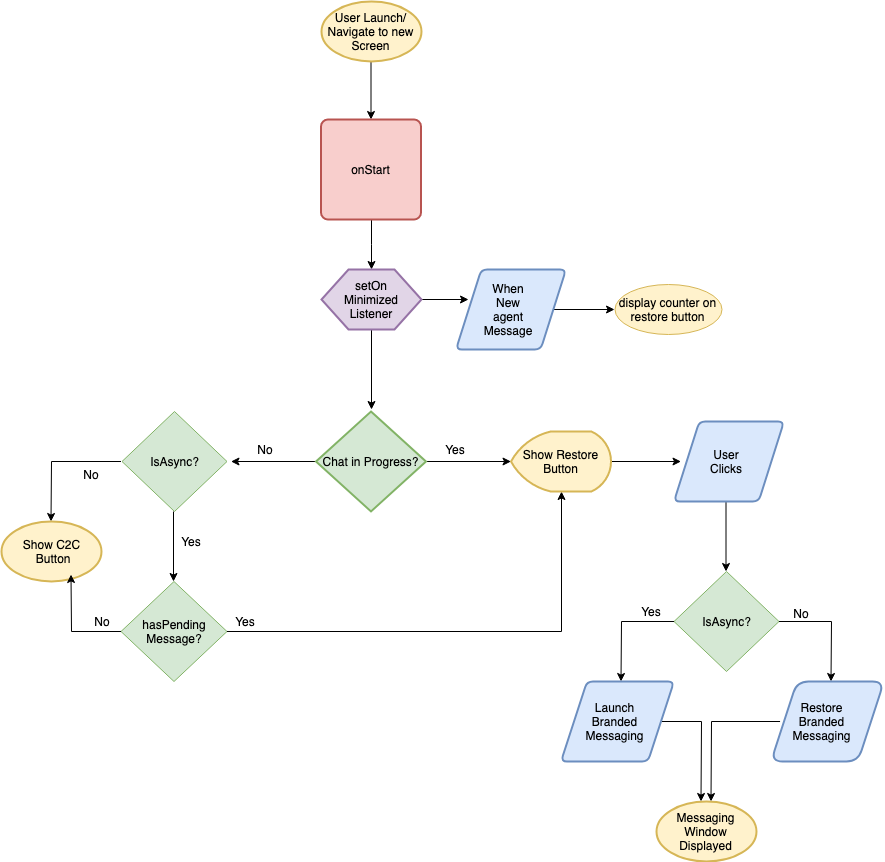
Check List
- Do you have clientID, clientSecret, Datacenter and tagserver values from Nuance?
- Do you have the engage parameters that is needed to start an engagement?
- Does your SDK timeout is same as the chatrouter timeout?
- Does user have a way to restore the messaging window upon minimized?
- Did you follow the previous Data Flow diagram when designing minimize/restore usecase.
- Does user is provided with a feedback when there is a new message from agent while Messaging window is minimized?
- Do you know the code that must be added to receive new agent message while minimized?
- Did you have a check for isChatInProgress before offering c2c button?
- Did you have a check for isChatInProgress when app comes back to foreground from background state?